Use Rivet component browser events
Learn how to work with browser events emitted by Rivet components
What you'll learn
- What component browser events are
- Why component browser events are useful
- How to set up event listeners for component browser events
- How to get a component browser event's target and details
- How to cancel a component browser event
What are component browser events? #
Component browser events are Event objects that are emitted when the user interacts with a JavaScript-powered Rivet component.
You can add event listeners for component browser events the same way you would for native browser events, such as click
.
The documentation for each JavaScript-powered component lists the browser events that it emits.
Why are component browser events useful? #
Component browser events are useful when you need to:
- Run code after the user interacts with a component in a specific way, such as closing a dialog
- Prevent component behaviors, such as opening and closing, in certain situations
1. Go to the accordion component page #
Go to the accordion component documentation.
Scroll down to the JavaScript section to see a table listing the browser events emitted by the accordion.
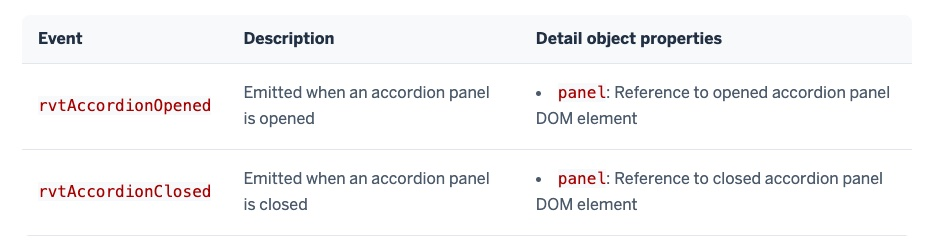
The browser events table has three columns:
- Event: The name of the browser event. Note that each event name starts with the prefix
rvt
. - Description: A brief description of the browser event.
- Detail object properties: A list of JavaScript properties on the
event.detail
object. This object contains additional context about the event, such as the specific accordion panel that was opened or closed. (You’ll learn more about thedetail
object later in this tutorial.)
2. Open the accordion example in CodePen #
Scroll up to the Example section on the accordion documentation page.
Click the Edit on CodePen button. This will open the accordion component in CodePen, an in-browser editor in which you can write HTML, CSS, and JavaScript.
3. Add a listener for the rvtAccordionOpened event #
You can use addEventListener()
to listen for Rivet component browser events in the same way you would listen for native browser events like click
.
The accordion component emits two browser events: rvtAccordionOpened
and rvtAccordionClosed
.
In this step, you’ll write code to show an alert when an accordion panel is opened.
3.1. Add empty event listener code #
First, add the following code to the JS panel in CodePen after Rivet.init()
:
document.addEventListener('rvtAccordionOpened', function (event) {
// Do something
})
This code instructs the browser to run the given function any time an accordion panel is opened anywhere in the document.
3.2. Show an alert when an accordion panel is opened #
Next, replace the // Do something
comment with the following line of code:
alert('Accordion panel opened')
Opening any of the accordion’s panels in the preview pane should now show an alert message:
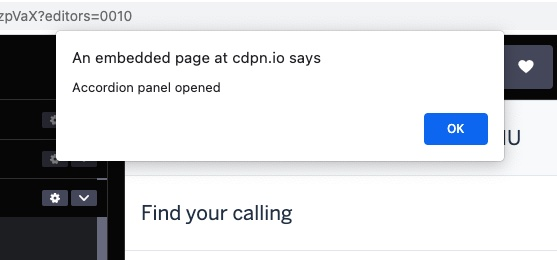
4. Inspect the event’s target and detail properties #
The event
object passed to the listener function has a target
property that contains a reference to the component’s root DOM element.
In some cases, the event
object also has a detail
property, which provides additional context about the event.
In this step, you’ll write code to inspect a Rivet component browser event’s target
and detail
properties.
4.1. Log the event target to the console #
First, replace the alert()
line in your JavaScript code with console.log(event.target)
:
document.addEventListener('rvtAccordionOpened', function (event) {
console.log(event.target)
})
4.2. Open the CodePen console #
Next, click on the Console button at the bottom-left of the CodePen interface. Doing so will show the JavaScript console pane.
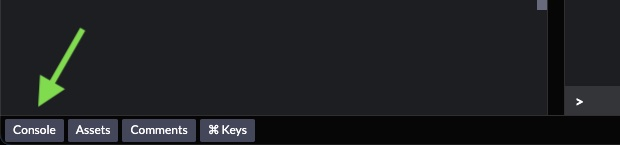
4.3. Open an accordion panel #
Open one of the accordion panels. You should see the markup for the accordion component DOM element printed to the console.
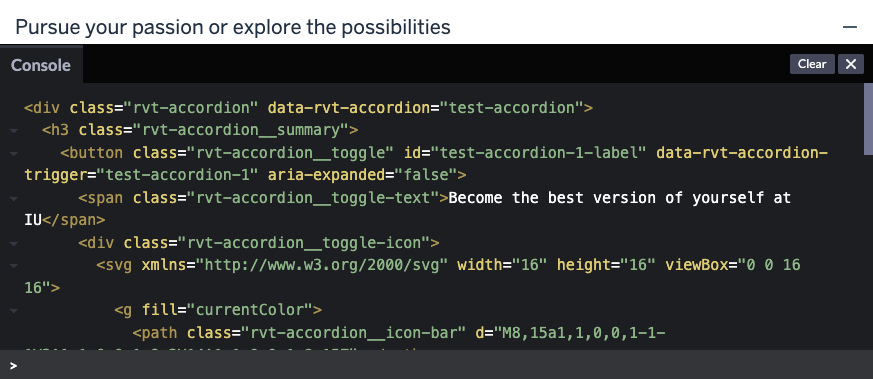
4.4. Log the opened panel to the console #
The rvtAccordionOpened
event’s detail
object has a property named panel
that contains a reference to the opened panel’s DOM element.
Replace event.target
with event.detail.panel
as shown below:
document.addEventListener('rvtAccordionOpened', function (event) {
console.log(event.detail.panel)
})
Opening a panel should now print that panel element’s markup to the console:
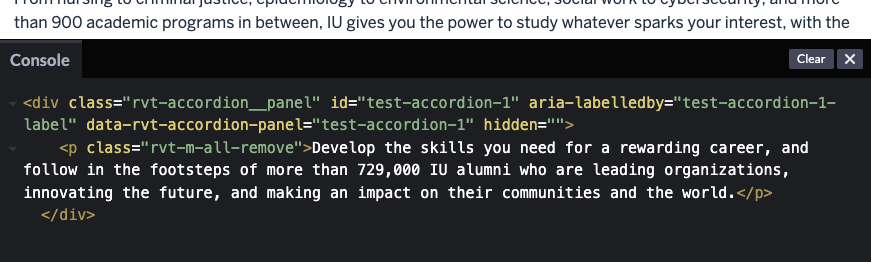
5. Cancel the event #
You can use event.preventDefault()
to cancel a Rivet component browser event.
Canceling a component event prevents that specific behavior from happening. For example, cancelling a rvtAccordionOpened
event stops the clicked panel from opening.
To see this behavior, update your event listener code as shown below:
document.addEventListener('rvtAccordionOpened', function (event) {
event.preventDefault()
})
Now, you should be unable to open any accordion panels in the preview pane.
6. Add a conditional statement around event.preventDefault() #
It’s not often that you’ll want to cancel all component events of a certain type all the time. It’s more likely that you’ll want to cancel a component event in specific situations or when your application is in a particular state.
In this step, you’ll add code to prevent the rvtAccordionOpened
event only if a specific panel is opened.
6.1. Get the opened accordion panel’s unique ID #
First, add the following lines to the start of your event listener function, before event.preventDefault()
:
const panel = event.detail.panel
const panelId = panel.dataset.rvtAccordionPanel
Recall from step 4 that the event.detail
property provides additional context about a Rivet component browser event.
In the case of the accordion, event.detail
has a panel
property that contains a reference to the opened accordion panel’s DOM element.
The second line in the code above reads the panel element’s dataset
property and saves the panel’s unique identifier to a variable called panelId
.
6.2. Check the opened panel’s ID before canceling the event #
The second panel in the example accordion has an ID of test-accordion-2
.
Add an if
statement to your event listener code that checks if the ID of the opened panel is test-accordion-2
. If so, run event.preventDefault()
.
document.addEventListener('rvtAccordionOpened', function (event) {
const panel = event.detail.panel
const panelId = panel.dataset.rvtAccordionPanel
if (panelId == 'test-accordion-2') {
event.preventDefault()
}
})
Now, only the second accordion panel should be prevented from opening.
Tutorial complete #
Congratulations—you have just learned how to work with component browser events! 🎉
Some things to try next:
- Use CodePen to experiment with other components’ browser events
- Learn more about using Rivet JavaScript